Hello! In this tutorial, I going to teach how to make a simple game using HTML, JavaScript, and CSS.
- Google Snake Game Html Code
- Snake And Ladder Game Html Code
- Simple Html Game Code
- Html5 Snake Code
- Snake Game Html Code Pdf
Here we are going to make a simple 8-bit Snake Game.
The important point is that our snake is formed by a chain of small squares.
In this tutorial, we will use the HTML canvas tag for developing this game, with Javascript code controlling the gameplay and the visuals of the game like the snake and the apple, growing of snake, etc. Following is the HTML code where we have used the tag to setup the game UI. Though, this game is usually played as a dual player. The current implementation is a single player and is more intended to show the concepts used and the potential behind it. Before I actually start explaining the code and implementation, I would like to touch the background by explaining the HTML Canvas element which is heart of the game.
The snake is moving with the help of an illusion wherein the last square is brought to the front.
This is built using a module pattern for code structure.

I am using Sublime Text for editing, you can use any editor of your wish.
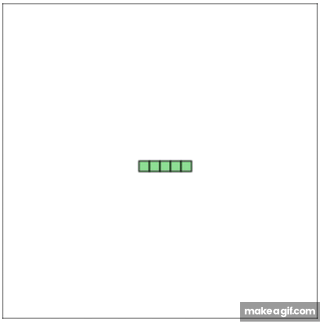
Also read: 3D Photo/Image Gallery (on space) Using HTML5 CSS JS
How to make a simple game using HTML
Let’s begin by creating a canvas element first.
Proceed by creating a button.
Follow the code for the procedure.
I have created a canvas of id=’canvas1′.
For button:-
Now I have added instructions for the game.
Now we make the settings javascript file for our game.
Google Snake Game Html Code
The getElementById() method returns the element that has the ID attribute with the specified value.
The variable ctx stores the content of the canvas
Create some global variables.
The parameter of getContext() tells that canvas is in the 2d plane.
Snake And Ladder Game Html Code
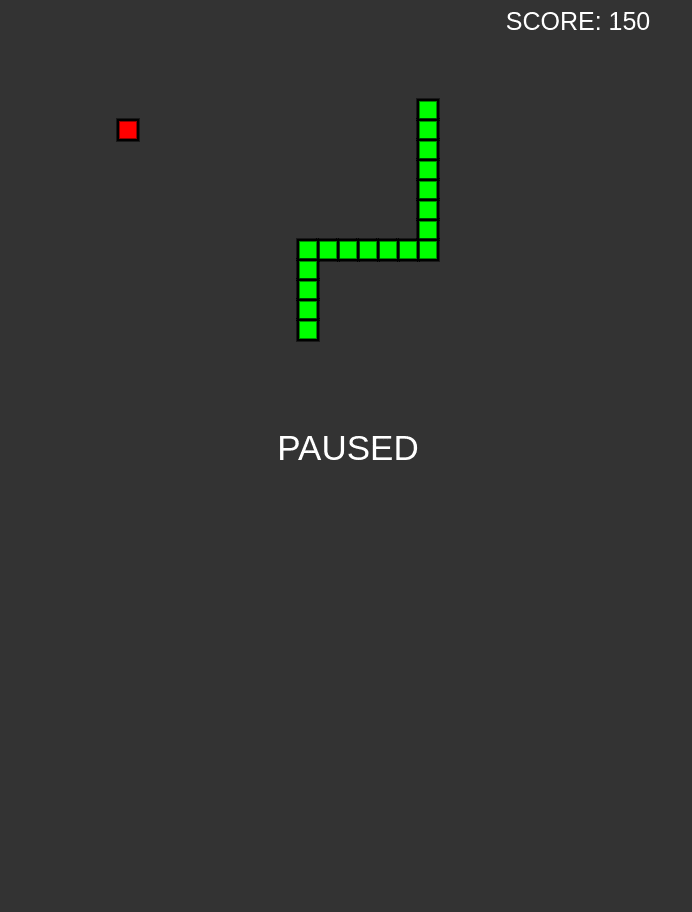
Now to draw the elements in canvas, create a javascript file and name it drawing.
Let’s draw the snake and its food.
The scoreText() function keeps the track of the score.
The cookie() function creates the food for the snake.
The bodySnake() function creates the head of the snake.
The above function creates the structure of the snake for our game. Initially, the length of the snake is 8 squares.
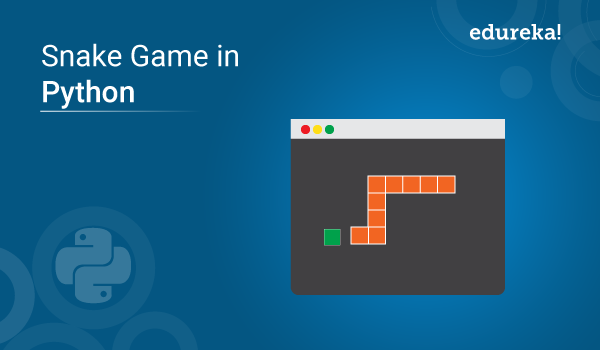
Create the structure of the food at random places inside the canvas using the following function:-
Now, we have to check whether the snake collides with itself or not.

Its time for the main function of our game.
Now, in the end we call the inint() function.
Don’t forget to close your module
Now, to add the controls we create a new file called application.js
The onekeydown event occurs when the user presses the arrow key.
The key bindings for the arrow keys are 37,38,39,40.
If the snake is facing left, then it cannot directly turn right because it would collide with itself.
We start by using a self-invoking anonymous function with drawModule() as a parameter.
Next, link your javascript file to the HTML document.
To add styling to your page use CSS.
I hope you enjoyed this tutorial.
Simple Html Game Code
Go ahead and make your own game and play!
Also, read JavaScript mousemove Event | Execute on moving the mouse pointer
Leave a Reply
- <html>
- <title></title>
- html, body {
- margin: 0;
- body {
- display: flex;
- justify-content: center;
- canvas {
- }
- </head>
- <canvas width='400' height='400'></canvas>
- var canvas = document.getElementById('game');
- var grid = 16;
- x: 160,
- // snake velocity. moves one grid length every frame in either the x or y direction
- dy: 0,
- // keep track of all grids the snake body occupies
- // length of the snake. grows when eating an apple
- };
- x: 320,
- };
- // @see https://stackoverflow.com/a/1527820/2124254
- return Math.floor(Math.random() * (max - min)) + min;
- // game loop
- requestAnimationFrame(loop);
- // slow game loop to 15 fps instead of 60 (60/15 = 4)
- return;
- count = 0;
- context.clearRect(0,0,canvas.width,canvas.height);
- snake.x += snake.dx;
- // wrap snake position horizontally on edge of screen
- snake.x = canvas.width - grid;
- else if (snake.x >= canvas.width) {
- }
- // wrap snake position vertically on edge of screen
- snake.y = canvas.height - grid;
- else if (snake.y >= canvas.height) {
- }
- // keep track of where snake has been. front of the array is always the head
- // remove cells as we move away from them
- snake.cells.pop();
- // draw apple
- context.fillRect(apple.x, apple.y, grid-1, grid-1);
- context.fillStyle = 'green';
- // drawing 1 px smaller than the grid creates a grid effect in the snake body so you can see how long it is
- context.fillRect(cell.x, cell.y, grid-1, grid-1);
- if (cell.x apple.x && cell.y apple.y) {
- // canvas is 400x400 which is 25x25 grids
- apple.y = getRandomInt(0, 25) * grid;
- // check collision with all cells after this one (modified bubble sort)
- for (var i = index + 1; i < snake.cells.length; i++) {
- // snake occupies same space as a body part. reset game
- if (cell.x snake.cells[i].x && cell.y snake.cells[i].y) {
- snake.y = 160;
- snake.maxCells = 4;
- snake.dy = 0;
- apple.y = getRandomInt(0, 25) * grid;
- }
- }
- document.addEventListener('keydown', function(e) {
- // prevent snake from backtracking on itself by checking that it's
- // not already moving on the same axis (pressing left while moving
- // left won't do anything, and pressing right while moving left
- // shouldn't let you collide with your own body)
- // left arrow key
- snake.dx = -grid;
- }
- else if (e.which 38 && snake.dy 0) {
- snake.dx = 0;
- // right arrow key
- snake.dx = grid;
- }
- else if (e.which 40 && snake.dy 0) {
- snake.dx = 0;
- });
- requestAnimationFrame(loop);
- </body>
Html5 Snake Code
Snake Game Html Code Pdf
